So, I've been doing a lot of reading, and I think I've finally come to the conclusion as to what my favorite JavaScript construction pattern is.
And the winner is...
I like this pattern more than this pattern:
The reason is because I feel like the first one is a little more easy to read.
It just seems a little more intuitive to me.
The conventions I use may be a little weird to you, but I'll walk you through why I have seemingly random capitalization.
Most functions and variable names I do in camelCase:
I have all objects start with a capital letter.
I know, I know -- "technically everything's an object".
To refine my previous statement, anything that's an object literal or an object created by a constructor.
Anything else, I do regular camelCase.
I find it allows me to recognize quickly where my objects are.
I'd be interested in hearing about your favorite JS patterns.
About Me
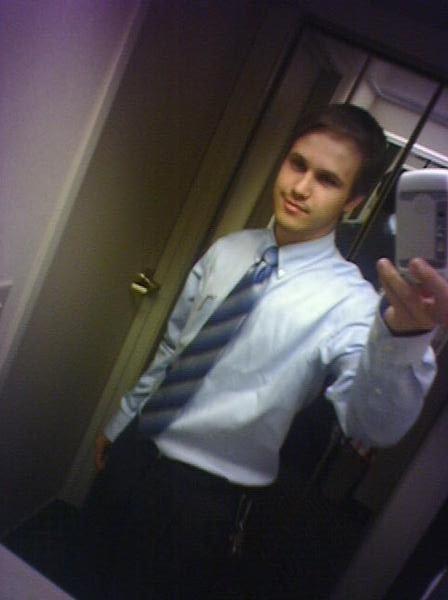
- Matthew Maxwell
- Experienced Web Developer using C#, ASP Classic (VBScript) and ASP.NET, MySQL, T-SQL, and other SQL variants, JavaScript (W3Schools Certified and very well versed in jQuery and learning Dojo), and XML. Heavy interest in JavaScript, framework creation on various language platforms, and keeping up with the best industry-accepted practices.
Wednesday, March 10, 2010
Monday, March 8, 2010
New conventions at work...
It doesn't take the smartest man in the world to know that without a good set of standards and best practices, a team of developers can have a real mess on their hands when it comes to project development and maintenance.
Last weekend, I got the privilege of being the coauthor of my team's set of best practices and coding conventions.
I am a relatively decent JavaScript developer, so I got to write the JavaScript portion of the document. I also contributed to the ASP and HTML/XML portions of the document as well.
I am pretty excited about the upcoming release of our document, as it will really help push our team to the next level, and give us scalable, maintainable code.
We've agreed to convert any page that needs edits over to the new set of standards before rerolling it to production.
This will give us some development time increases, but in the long run, the benefit is just priceless!
Last weekend, I got the privilege of being the coauthor of my team's set of best practices and coding conventions.
I am a relatively decent JavaScript developer, so I got to write the JavaScript portion of the document. I also contributed to the ASP and HTML/XML portions of the document as well.
I am pretty excited about the upcoming release of our document, as it will really help push our team to the next level, and give us scalable, maintainable code.
We've agreed to convert any page that needs edits over to the new set of standards before rerolling it to production.
This will give us some development time increases, but in the long run, the benefit is just priceless!
Thursday, February 11, 2010
Optimizing your jQuery code - fragment caching rules
jQuery 1.4 really introduced a lot of cool shit. One thing in particular is the internal caching of elements. When used properly, this can drastically increase your application's performance!
A good example is one I'm currently doing. Currently, I'm working on an admin page that allows a manager to go in and add/edit/remove options, details, and other data that are used by another application front-end for our tech support partners.
I'm doing the modification via AJAX, so when they click on an option, it will grab all the details for that option, and display them, so they can be edited. I'm caching everything grabbed and holding it locally, so I only have to do one AJAX call per option.
When they click the edit button, I'm having the innerHTML of the cells of that row turn into input fields for them to type the new, edited data, and then save when they hit update, reverting back to a regular text-filled cell.
As you can tell, I will be inserting a lot of inputs.
jQuery 1.4 introduced a new way to add elements to your page.
The old way (1.3.2) was something like
or
Something like that.
Now with jQuery 1.4, you can do
This is similar to the second example, the object passed as the second parameter is an attribute object.
What makes 1.4 really awesome, though is that if you use the method of $("<input>") either with an attribute object or going $("<input>").attr(), it will cache the fragments it creates when making these.
So, when you're adding multiple elements, you're basically getting a clone of the cached fragment, and not having to recreate an entirely new element.
The speed and performance boost from this is crazy, and I'd definitely recommend messing around with it.
A good example is one I'm currently doing. Currently, I'm working on an admin page that allows a manager to go in and add/edit/remove options, details, and other data that are used by another application front-end for our tech support partners.
I'm doing the modification via AJAX, so when they click on an option, it will grab all the details for that option, and display them, so they can be edited. I'm caching everything grabbed and holding it locally, so I only have to do one AJAX call per option.
When they click the edit button, I'm having the innerHTML of the cells of that row turn into input fields for them to type the new, edited data, and then save when they hit update, reverting back to a regular text-filled cell.
As you can tell, I will be inserting a lot of inputs.
jQuery 1.4 introduced a new way to add elements to your page.
The old way (1.3.2) was something like
or
Something like that.
Now with jQuery 1.4, you can do
This is similar to the second example, the object passed as the second parameter is an attribute object.
What makes 1.4 really awesome, though is that if you use the method of $("<input>") either with an attribute object or going $("<input>").attr(), it will cache the fragments it creates when making these.
So, when you're adding multiple elements, you're basically getting a clone of the cached fragment, and not having to recreate an entirely new element.
The speed and performance boost from this is crazy, and I'd definitely recommend messing around with it.
Labels:
jquery,
optimization
Wednesday, February 10, 2010
Tuesday, January 26, 2010
Ternary Operation in JavaScript
Ternary operations in JavaScript are really awesome.
It's essentially an if/then block, but on one line.
An example would be:
In this example, y would be "howdy".
You can read ternary operations as
y = ( comparison ) ? if it's true, use this value : otherwise, use this value ;
This is a very simple example, but you can see the power behind this.
An example in jQuery could be...
Again, this is a very simple example, but you get the point.
One word of advice, though: never use ternary operations if the values to be set are true/false:
Instead, just use the comparison:
It's essentially an if/then block, but on one line.
An example would be:
In this example, y would be "howdy".
You can read ternary operations as
y = ( comparison ) ? if it's true, use this value : otherwise, use this value ;
This is a very simple example, but you can see the power behind this.
An example in jQuery could be...
Again, this is a very simple example, but you get the point.
One word of advice, though: never use ternary operations if the values to be set are true/false:
Instead, just use the comparison:
Labels:
beginning javascript,
javascript
Monday, January 25, 2010
jQuery 1.4 and native JSON support
I was reading a post by Yehuda Katz (http://www.twitter.com/wycats), and saw how jQuery 1.4 now uses native JSON support, which is available in a lot of more current browsers (IE8, FF 3.5, Chrome 2), and when JSON support is not available, it falls back on its previous JSON parsing.
In a video by Paul Irish (http://www.twitter.com/paul_irish), he states that jQuery 1.4 will now validate the JSON (if native JSON support is not available) and throw errors if the JSON response is not valid JSON, just as native JSON parse would throw errors.
Both of these things are awesome. I am pretty excited about that.
I have been guilty of using malformed JSON simply because I could get away with it.
Now, a great standard is being pushed to the forefront!
I am going to be taking it a step further and ensure that all the JSON I use throughout my apps are valid JSON (even objects I'm passing to jQuery functions).
I'm doing this for a few reasons. One reason is consistency. I like having consistent organization and standards in my applications. This is a must at my job because different members of the team may have to go in and edit my code. If I have a consistent style of programming, they'll be able to easily go in and make changes.
Another reason is, it's just good practice. If I do ALL my JSON in valid JSON format, then I won't even have to go "oh, this is an AJAX call, my response should be valid JSON", I'll just be doing ALL my JSON in valid format, and not have to worry about it.
What are your thoughts about this?
In a video by Paul Irish (http://www.twitter.com/paul_irish), he states that jQuery 1.4 will now validate the JSON (if native JSON support is not available) and throw errors if the JSON response is not valid JSON, just as native JSON parse would throw errors.
Both of these things are awesome. I am pretty excited about that.
I have been guilty of using malformed JSON simply because I could get away with it.
Now, a great standard is being pushed to the forefront!
I am going to be taking it a step further and ensure that all the JSON I use throughout my apps are valid JSON (even objects I'm passing to jQuery functions).
I'm doing this for a few reasons. One reason is consistency. I like having consistent organization and standards in my applications. This is a must at my job because different members of the team may have to go in and edit my code. If I have a consistent style of programming, they'll be able to easily go in and make changes.
Another reason is, it's just good practice. If I do ALL my JSON in valid JSON format, then I won't even have to go "oh, this is an AJAX call, my response should be valid JSON", I'll just be doing ALL my JSON in valid format, and not have to worry about it.
What are your thoughts about this?
Labels:
jQuery 1.4,
JSON
Friday, January 15, 2010
JavaScript console
At my company, I am required to support different browsers because a lot of employees here like to use their preferred browser; we support IE6+, FF2+, and Chrome. Let me start by saying I love Chrome and I love FireFox. They both have different aspects about them that are amazing.
I love Chrome because it's just really good quality. Google has never really produced any bad products to my knowledge. They're always working to be on the cutting edge and they do a damn good job at it. The V8 engine in Chrome is fan-effing-tastic.
Firefox is a well-rounded browser that has the ability to be customized out the ying-yang. It's Firebug plugin is absolutely amazing. There are also some other plugins I use that are really cool.
One thing that make Chrome and Firefox even better is the fact that they both adhere to web standards! What a concept, right? Haha.
Another thing I love about them is the fact that they have a JavaScript console, which has proved to be a priceless commodity.
IE, however, does not...unless you install something like debugbar (which is a very heavy IE plugin).
I took some time and actually created a console (written completely in JavaScript) that works across all browsers. I can throw different commands at the console, and even have a mode set up to take and interpret JavaScript commands. I use this console in a newer application I'm going to release as an actual event log for my app. It's pretty light weight, and I have added the ability for it to email my team a timestamped log of all events/responses from the application.
I have it self-truncating at 500 rows currently, just to ensure the log doesn't get overly massive.
I just bought my own website (www.matthewcmaxwell.com) and will be setting that up in the near future. When I do, I'll be putting my JavaScript console up there for you to download and try out.
Here's a small pic (bad quality, I know)
I love Chrome because it's just really good quality. Google has never really produced any bad products to my knowledge. They're always working to be on the cutting edge and they do a damn good job at it. The V8 engine in Chrome is fan-effing-tastic.
Firefox is a well-rounded browser that has the ability to be customized out the ying-yang. It's Firebug plugin is absolutely amazing. There are also some other plugins I use that are really cool.
One thing that make Chrome and Firefox even better is the fact that they both adhere to web standards! What a concept, right? Haha.
Another thing I love about them is the fact that they have a JavaScript console, which has proved to be a priceless commodity.
IE, however, does not...unless you install something like debugbar (which is a very heavy IE plugin).
I took some time and actually created a console (written completely in JavaScript) that works across all browsers. I can throw different commands at the console, and even have a mode set up to take and interpret JavaScript commands. I use this console in a newer application I'm going to release as an actual event log for my app. It's pretty light weight, and I have added the ability for it to email my team a timestamped log of all events/responses from the application.
I have it self-truncating at 500 rows currently, just to ensure the log doesn't get overly massive.
I just bought my own website (www.matthewcmaxwell.com) and will be setting that up in the near future. When I do, I'll be putting my JavaScript console up there for you to download and try out.
Here's a small pic (bad quality, I know)

Subscribe to:
Posts (Atom)